Customize search results
Available features and filters can be applied to customize the order of results returned by Enterprise Search.
Sorting by date
Note
If search results are being returned in an unexpected order, they are probably being sorted by WP_Query
in the application’s code. In order for search results to be returned by Elasticsearch’s default ordering by relevancy, locate and remove the sorting from WP_Query
.
Use the ep_set_sort
filter to sort Elasticsearch results by date.
For example, to sort from newest to oldest:
add_filter( 'ep_set_sort', 'ep_sort_by_date', 20, 2 );
function ep_sort_by_date( $sort, $order ) {
$sort = array(
array(
'post_date' => array(
'order' => $order,
),
),
);
return $sort;
}
Relevancy
If too many loosely relevant results are included at the end of a results list, use min_score
on the Elasticsearch query to trim them down without hurting the more relevant results. A good starting point depends on your scoring methods; review the max_score
and individual _score
values in the response JSON using Search Dev Tools to determine what works for you.
For example, by modifying min_score
and max_score
, a search for the term “import” will return fewer results that match the term “port”.
In this code example, the min_score
is based on the scores obtained on a simple test site, without additional customizations.
add_filter( 'ep_formatted_args', 'vip_sample_filter_min_score', 25, 3 );
/**
* filter the min_score to exclude unrelated results
*
*/
function vip_sample_filter_min_score( $formatted_args, $args, $wp_query ) {
/**
* adjust min_score
*
* higher score = better matches
* lower score = looser matching, more results
*/
$formatted_args['min_score'] = 0.002;
return $formatted_args;
}
The assigned min_score
value is arbitrary and can be dependent on the search terms themselves.
Fuzziness
Use fuzziness to return results that account for terms that are similar to the term that was searched for.
Fuzziness is enabled by:
- Updating the search algorithm version to
4.0
with the addedep_search_algorithm_version
filter. - Setting the level of fuzziness (measured by Levenshtein distance) with the
ep_fuzziness_arg
filter.
In this code example, fuzziness is enabled and set to a level of 1
:
add_filter( 'ep_search_algorithm_version', 'set_search_algorithm_version' );
/**
* Set algorithm version to 4.0 required to enable fuzziness search
*/
function set_search_algorithm_version() {
return '4.0';
}
add_filter( 'ep_fuzziness_arg', 'set_fuzziness' );
/**
*
*/
function set_fuzziness() {
return 1; // increase as needed to increase the fuzziness span
}
Aggregation
Aggregation is a method for Elasticsearch to group documents into buckets based on criteria.
By default, no taxonomies are configured for aggregation. Customizing aggregations requires the facets feature to be enabled.
Including taxonomy types
The ep_facet_include_taxonomies
filter is used to select the taxonomies to include in faceting.
Note
The ep_facet_include_taxonomies
filter will add term aggregations to every offloaded Elasticsearch query, which can negatively affect query performance. Adding a condition is recommended to improve performance, unless the ep_facet_include_taxonomies
filter is being applied to a widget.
In this code example, the category
taxonomy is included in faceting:
add_filter( 'ep_facet_include_taxonomies', 'vip_taxonomy_facets_included' );
function vip_taxonomy_facets_included( $taxonomies ) {
$category = get_taxonomy( 'category' );
$taxonomies = array( 'category' => $category );
return $taxonomies;
}
Configuring taxonomy type size
When a taxonomy is included in faceting, the number (size) of the taxonomy’s groupings to be returned in the facet can be configured. By default the taxonomy type size is 5
, but can be customized with the ep_facet_taxonomies_size
filter.
Caution
Taxonomy type size values have an effect performance. Smaller taxonomy type size values scale better.
In this code example, the taxonomy type size is set to 10
for the category
taxonomy:
add_filter( 'ep_facet_taxonomies_size', 'vip_set_facet_taxonomies_size', 10, 2 );
function vip_set_facet_taxonomies_size( $size, $taxonomy ) {
if ( 'category' === $taxonomy->name ) {
$size = 10;
}
return $size;
}
Accessing aggregations
To access the aggregations, use the $ep_facet_aggs
global (only available at the wp
action and after):
function vip_get_aggs() {
global $wp_query;
if ( ! $wp_query->elasticsearch_success ) {
return; // Unsuccessful ES request, bail.
}
global $ep_facet_aggs;
if ( isset( $ep_facet_aggs ) ) {
// Do stuff.
}
}
Adding a date histogram
To add a date histogram as part of the aggregations, use the ep_formatted_args
filter.
In this code example, “year” is bucketed during search:
add_filter( 'ep_formatted_args', 'vip_add_date_histogram_to_aggs', 10, 3 );
function vip_add_date_histogram_to_aggs( $formatted_args, $args, $wp_query ) {
if ( ! is_search() ) {
return;
}
$year = array(
'filter' => $formatted_args['post_filter'],
'aggs' => array(
'years' => array(
'terms' => array(
'field' => 'date_terms.year',
'order' => array( '_key' => 'desc' ),
),
),
),
);
$formatted_args['aggs']['date_histogram'] = $year;
return $formatted_args;
}
Search Fields
To adjust what fields are being searched, use the WP_Query
argument of search_fields
. By default, these fields default to post_title
, post_excerpt
and post_content
.
In this code example, the pre_get_posts
hook is used to modify all search queries to only search across post_title
, taxonomies of category
and post_tag
, and meta of meta_key_1
:
add_action( 'pre_get_posts', 'vip_add_search_fields' );
function vip_add_search_fields( $query ) {
if ( $query->is_search() ) {
$search_fields = array(
'post_title',
'taxonomies' => array(
'category',
'post_tag',
),
'meta' => array(
'meta_key_1',
),
);
$query->set( 'search_fields', $search_fields );
}
}
Note
Passing in search_fields
will automatically disable any custom search weighting configurations.
Synonyms
Synonyms allows for associating search terms with each other, which can be done by either Sets or Alternatives.
Sets
Sets are terms that should equally match each other in search results. For example, the regional variation spelling of a color such as grey
versus gray
:
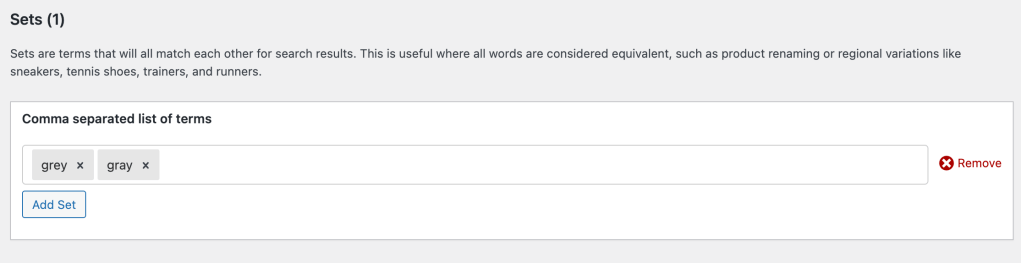
Alternatives
Alternatives consist of a primary term that will match other terms when you search for the primary term. For example, using the primary term of shoes
, alternatives can be listed as sneakers
, sandal
, boots
, high heels
:
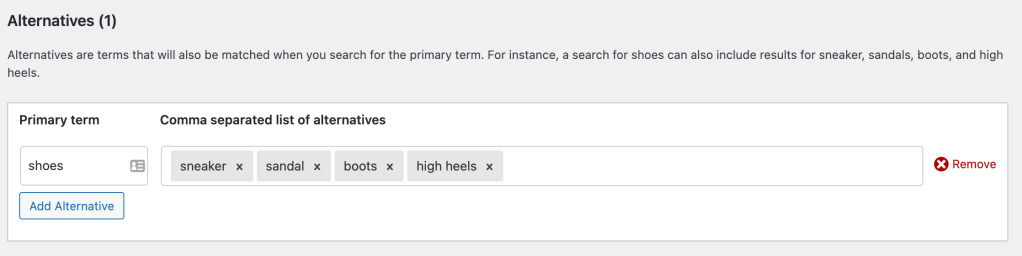
Advanced Text Editor
The Advanced Text Editor is available to use for editing synonyms directly, where it is useful for exporting or importing large amounts of synonyms.
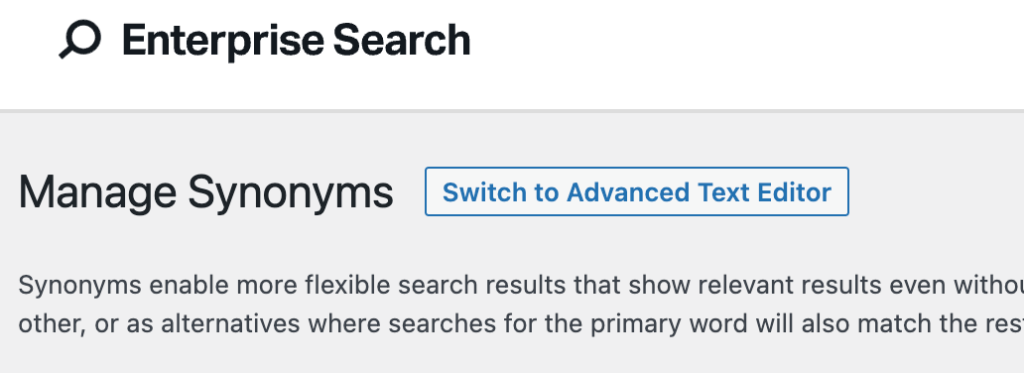
Sets are defined by terms separated out by commas and alternatives are defined by a key => value pairing:
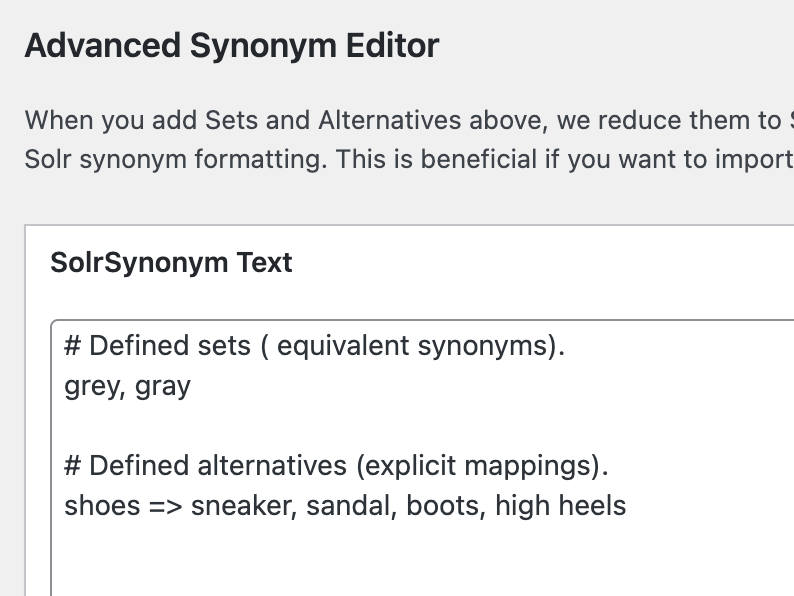
Last updated: May 26, 2025